Docusaurus: Side menu on custom page
I’ve been working with Docusaurus to build the dev.startree.ai website over the last few months and I wanted to add a custom page with a sidebar similar to the one that gets automatically generated on documentation pages.
All the examples I could find showed you to create a splash page, so it took me a while to figure out how to do what I wanted, but in this post we’ll learn how to do it.
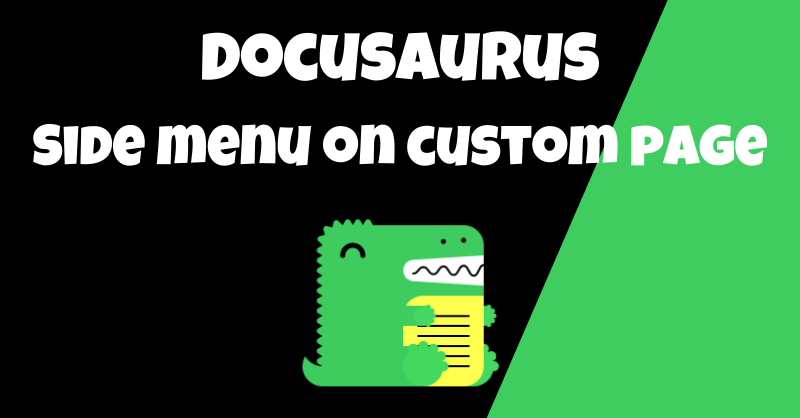
First, let’s create a Docusaurus site called my-website
by running the following command:
npx create-docusaurus@latest my-website classic
We can run the site locally with the following command:
npm run start
This will run a local webserver at http://localhost:3000, which will show the following page:
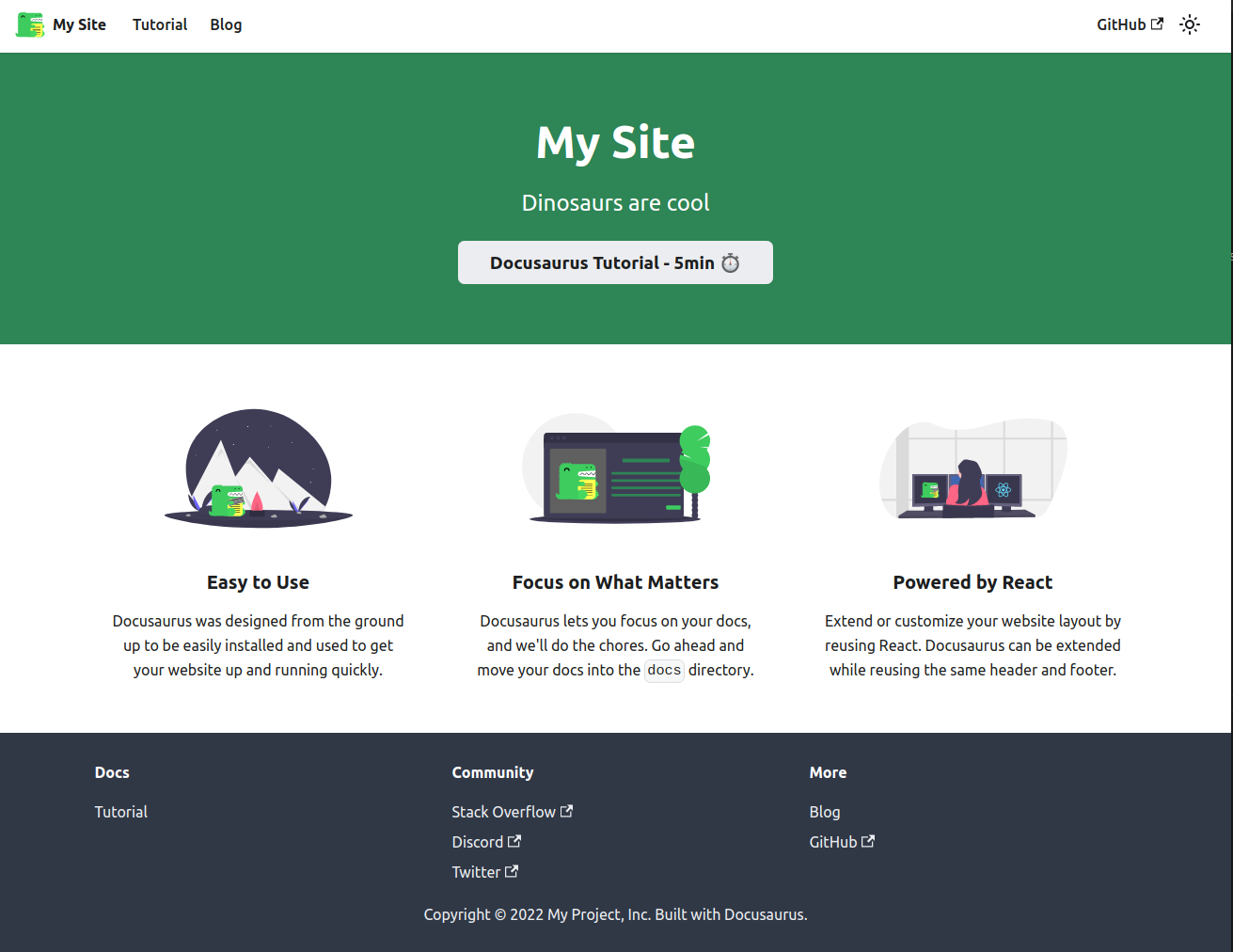
Custom pages go under src/pages
and whatever we name our file will be the name of the page.
Let’s create myCustomPage.js
with the following contents:
import React from 'react';
import clsx from 'clsx';
import Layout from '@theme/Layout';
import DocSidebar from '@theme/DocSidebar';
import { ThemeClassNames } from '@docusaurus/theme-common';
import MainStyles from '@docusaurus/theme-classic/lib/theme/DocPage/Layout/Main/styles.module.css';
import DocPageStyles from '@docusaurus/theme-classic/lib/theme/DocPage/Layout/styles.module.css';
import SidebarStyles from '@docusaurus/theme-classic/lib/theme/DocPage/Layout/Sidebar/styles.module.css';
import DocItemColStyles from '@docusaurus/theme-classic/lib/theme/DocItem/Layout/styles.module.css';
import DocItemStyles from '@docusaurus/theme-classic/lib/theme/TOC/styles.module.css';
import DocBreadcrumbs from '@docusaurus/theme-classic/lib/theme/DocBreadcrumbs/styles.module.css';
import MDXContent from '@theme/MDXContent';
import useDocusaurusContext from '@docusaurus/useDocusaurusContext';
export default function MyCustomPage() {
const { siteConfig } = useDocusaurusContext();
const name = "My Custom Page"
return (
<Layout
title={`My Custom Page | ${siteConfig.title}`}
>
<div className={DocPageStyles.docPage}>
<aside className={clsx(
ThemeClassNames.docs.docSidebarContainer,
SidebarStyles.docSidebarContainer
)}>
<DocSidebar sidebar={[
{ type: "link", href: "/myCustomPage", label: "My Custom Page" },
{ type: "link", href: "/anotherCustomPage", label: "Another Custom Page" }
]} path="/myCustomPage">
</DocSidebar>
</aside>
<main className={clsx(MainStyles.docMainContainer)}>
<div className={clsx('container', 'padding-top--md', 'padding-bottom--lg')}>
<div className="row">
<div className={clsx('col', DocItemColStyles.docItemCol)}>
<div className={DocItemStyles.docItemContainer}>
<article>
<nav className={DocBreadcrumbs.breadcrumbsContainer}>
<ul className="breadcrumbs">
<li class="breadcrumbs__item"><a aria-label="Home page" class="breadcrumbs__link" href="/"><svg viewBox="0 0 24 24" className={DocBreadcrumbs.breadcrumbHomeIcon}><path d="M10 19v-5h4v5c0 .55.45 1 1 1h3c.55 0 1-.45 1-1v-7h1.7c.46 0 .68-.57.33-.87L12.67 3.6c-.38-.34-.96-.34-1.34 0l-8.36 7.53c-.34.3-.13.87.33.87H5v7c0 .55.45 1 1 1h3c.55 0 1-.45 1-1z" fill="currentColor"></path></svg></a></li>
<li itemscope="" itemprop="itemListElement" itemtype="https://schema.org/ListItem" class="breadcrumbs__item breadcrumbs__item--active"><span class="breadcrumbs__link" itemprop="name">{name}</span><meta itemprop="position" content="2" /></li>
</ul>
</nav>
<div className={clsx(ThemeClassNames.docs.docMarkdown, 'markdown')}>
<MDXContent>
Our custom text goes here.
</MDXContent>
</div>
</article>
</div>
</div>
</div>
</div>
</main>
</div>
</Layout>
);
}
If we want to adjust the items show in the side bar we’d need to change the DocSidebar
component’s sidebar
property.
Most of the code shown here describes CSS styles that do change location between minor versions.
I’ll try to keep the example up to date with the latest version.
Note
|
The version of the docusaurus at the time of writing this blog post is 2.0.0-beta.22. |
The custom page is created at http://localhost:3000/myCustomPage and looks like this:
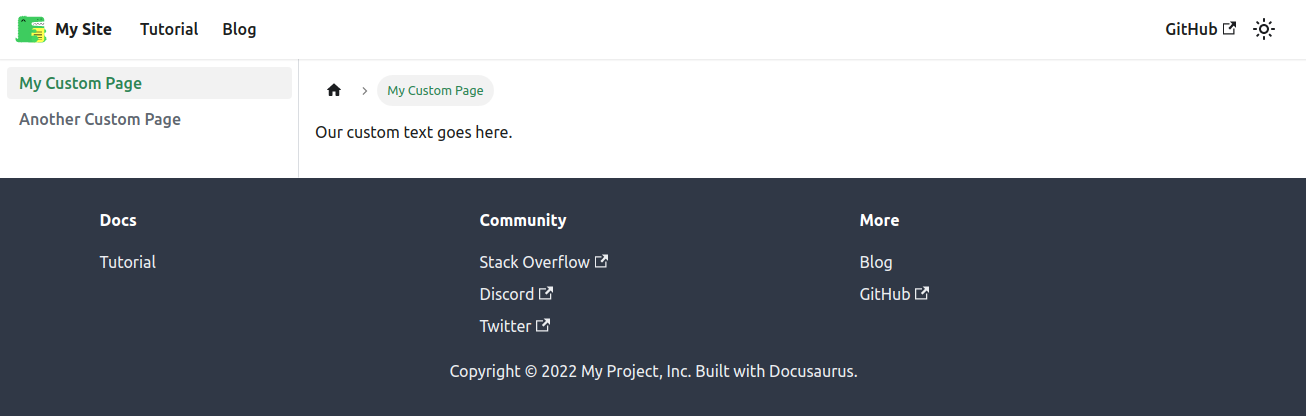
About the author
I'm currently working on short form content at ClickHouse. I publish short 5 minute videos showing how to solve data problems on YouTube @LearnDataWithMark. I previously worked on graph analytics at Neo4j, where I also co-authored the O'Reilly Graph Algorithms Book with Amy Hodler.